Data Visualization with Hand-Drawn/Sketchy Style
Data visualization can help to understand and analyze statistical data in a more intuitive way by graphing the data. In recent years, it is common to see companies using hand-drawn data visualization in user reports or blogs to make their content and style more relatable. This article lists some common data visualization tools and applicable charts.
Tools | rough + draw.io | matplotlib.pyplot.xkcd | chart.xkcd & cutecharts | instad.io |
---|---|---|---|---|
Scope | For existing draw.io charts, svg charts or charts that need to be drawn directly on the canvas | For data visualization charts, especially those generated by matplotlib or seaborn. Embedded in jupyter labs/notebooks | For data visualization charts with interactive requirements. Embedded in web pages or jupyter lab/notebooks | For existing svg or spreadsheet charts. Can be converted directly to hand-drawn style |
Charts | Any chart, especially for direct diagrams such as flowcharts, class charts or timeline charts, etc. | Suitable for most data visualization charts, such as line, bar, pie, contour, etc. | Only supports 'bar', 'line', 'pie', 'radar', 'scatter' | Any chart, only requires DOM input format SVG or PDF charts |
Draw Diagram with Hand-Drawn Style
rough + draw.io
rough is a very powerful hand-drawn style base tool for implementing basic drawing elements in hand-drawn style on Canvas and SVG. rough is a very small js library (~9KB) that defines basic units for drawing lines, curves , arcs, polygons, circles and ellipses as basic units. We can implement hand-drawn images on Canvas by defining some basic units on the js side, a simple example of which is shown below.
rough render code
<script src="https://unpkg.com/roughjs@latest/bundled/rough.js"></script> |
Because of its small size and ease of use, rough is embedded in many software to achieve hand-drawn style, such as draw.io, which I use a lot. draw.io is a free online diagram editor, which is light and feature-rich, and supports instant store and multi-person online editing. It is an ideal tool for study and work. draw.io supports hand-drawn style diagramming throughrough around 2020, and there is an official blog about this feature. In short, we first need to select the hand-drawn style (sketch) on the right side of the overall chart style, and if we need to fine-tune the hand-drawn style of each element, we need to select the corresponding one by the fill method and line of each element, or even change the sketch style by the property of the drawing unit. If text is needed, the font should be changed to Comic Sans MS. The simple process is shown in the figure below.
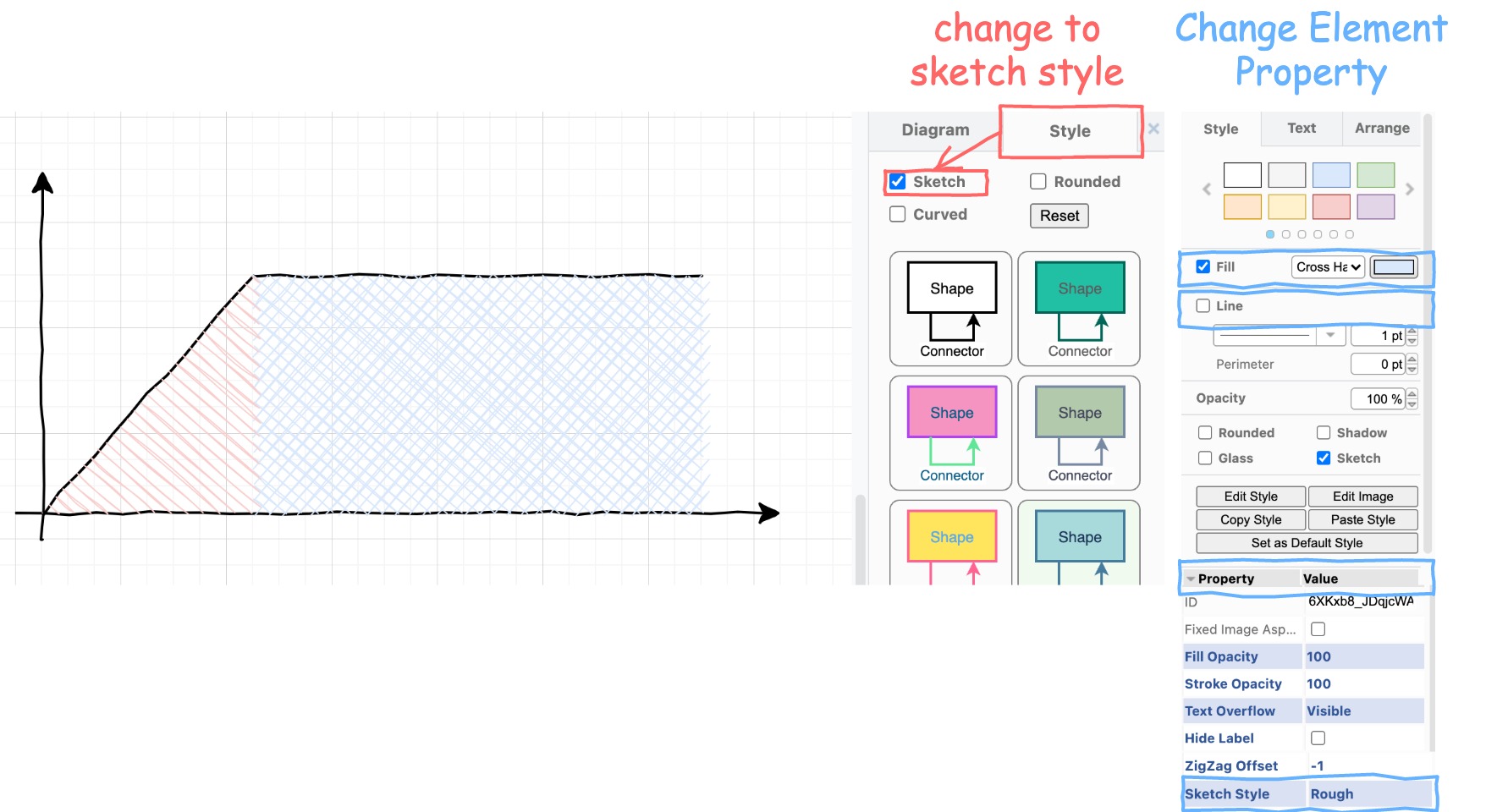
With draw.io, you can style most of the diagrams into a hand-drawn style, such as flowcharts.
matplotlib.pyplot.xkcd
Matplotlib is the most commonly used data visualization library in
python. Matplotlib itself also supports hand-drawn style. Matplotlib
loads and controls the hand-drawn style through its
matplotlib.pyplot.xkcd api. To use it, you just need to put the original
matplotlib plot inside of with matplotlib.pyplot.xkcd()
scope to convert the image to hand-drawn style. This method is also
applicable to the seaborn data visualization library as well, which is
based on matplotlib. An example is shown below, Figure 1 represents the
pie chart drawn by the original matplotlib, and Figure 2 represents the
pie chart converted to hand-drawn style by placing the original
matplotlib plot in with matplotlib.pyplot.xkcd()
.
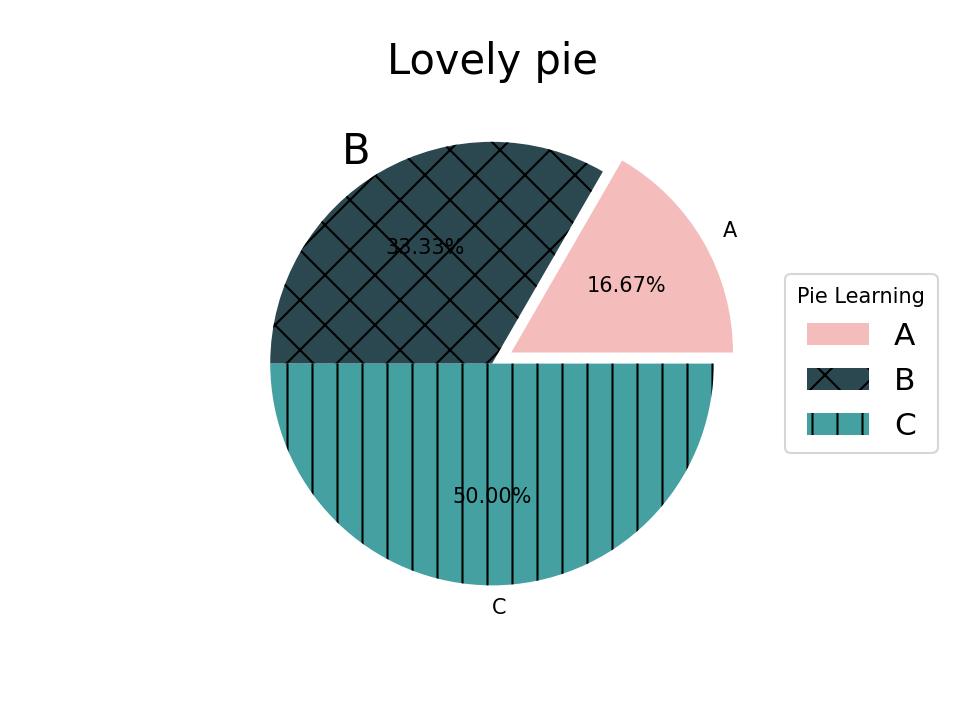
matplotlib original render code
import matplotlib.pyplot as plt |
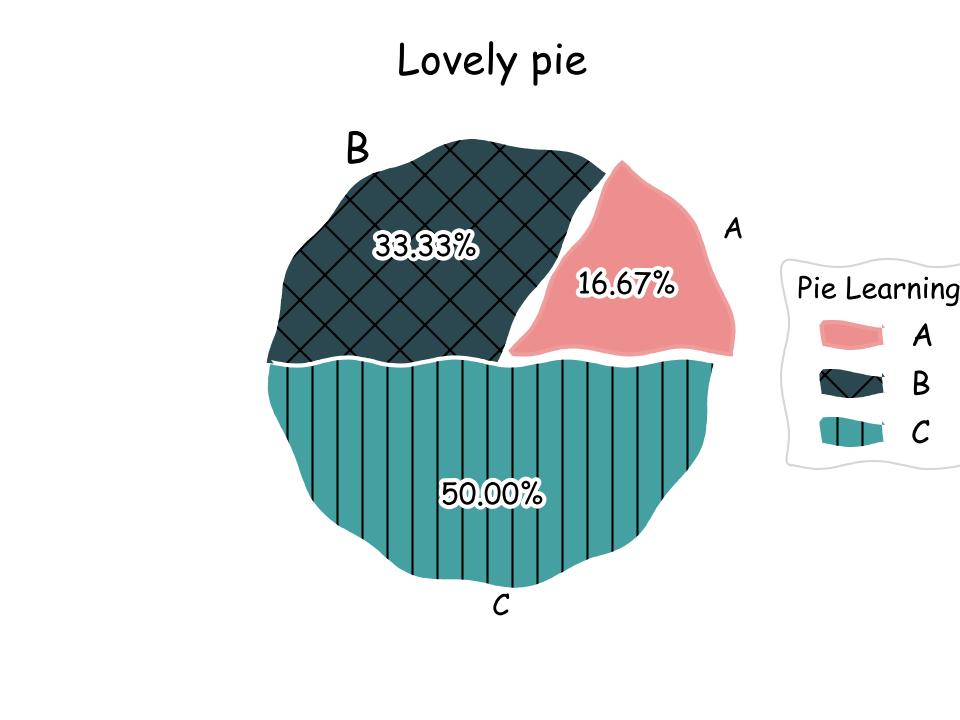
matplotlib.xkcd render code
import matplotlib.pyplot as plt |
When using matplotlib or seaborn for data visualization, this method makes it easy and convenient to convert patterns into a hand-drawn style.
Interactive Diagram with Hand-Drawn Style:chart.xkcd & cutecharts
When talking about hand-drawn style data visualization, chart.xkcd and cutecharts are probably two of the most popular tools.
chart.xkcd is a JavaScript library for interactive hand-drawn style data visualization, while cutecharts is a wrapper of chart.xkcd to python.
The hand-drawn style effect of chart.xkcd is shown below.
chart.xkcd render code
<script src="https://cdn.jsdelivr.net/npm/chart.xkcd@1.1/dist/chart.xkcd.min.js"></script> |
The api of cutecharts is very close to that of pyechart, and the example is shown below.
cutecharts render code
from cutecharts.charts import Bar |
Similar to pyechart, using bar_base().render()
will
generate the chart as an html file, i.e. the pattern rendered in the
above image, the generated code is as follows.
|
It is a pity that chart.xkcd and cutecharts have limited support for graph categories, only supporting 'bar', 'line', 'pie', 'radar', 'scatter'. Its api also does not support many features, such as auxiliary lines or additional annotations. And the developer has stopped development around 2019, so it is also hard to support different kinds of charts and functions in the foreseeable future. These limitations make it really hard to be comparable with plotly, echart or pyechart in the most usecases.
There are some other libraries which are similar to chart.xkcd and cutecharts: rough-charts and roughViz are JavaScript library based on rough and d3, and py-roughviz, a python library wrapped in roughViz also implements hand-drawn style data visualization. But its function is similar to chart.xkcd and cutecharts, the api is relatively complicated, as well as more or less stopped the development. Therefore, I don't introduce the detail of those tools, more reference could be found in the official documentation and examples.
Convert Diagram to Hand-Drawn Style:instad.io
In addition to the above methods, we can also convert existing svg (which can be generated by libraries such as pyechart or matplotlib or software such as draw.io, PS, AI, etc.) or spreadsheet charts by instad.io into hand-drawn style. The result is shown in the figure below. For a detailed example, please refer to instad.io.
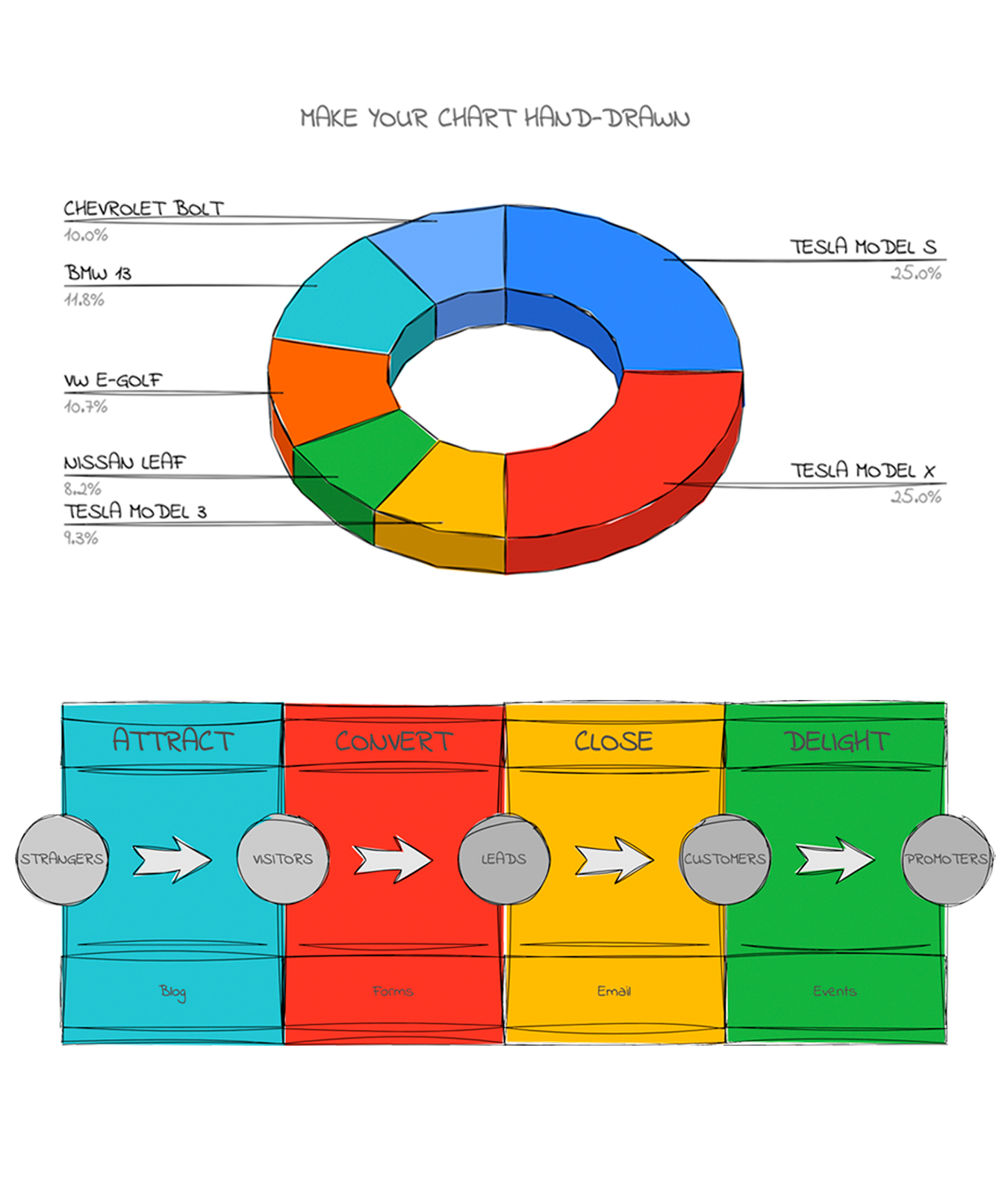
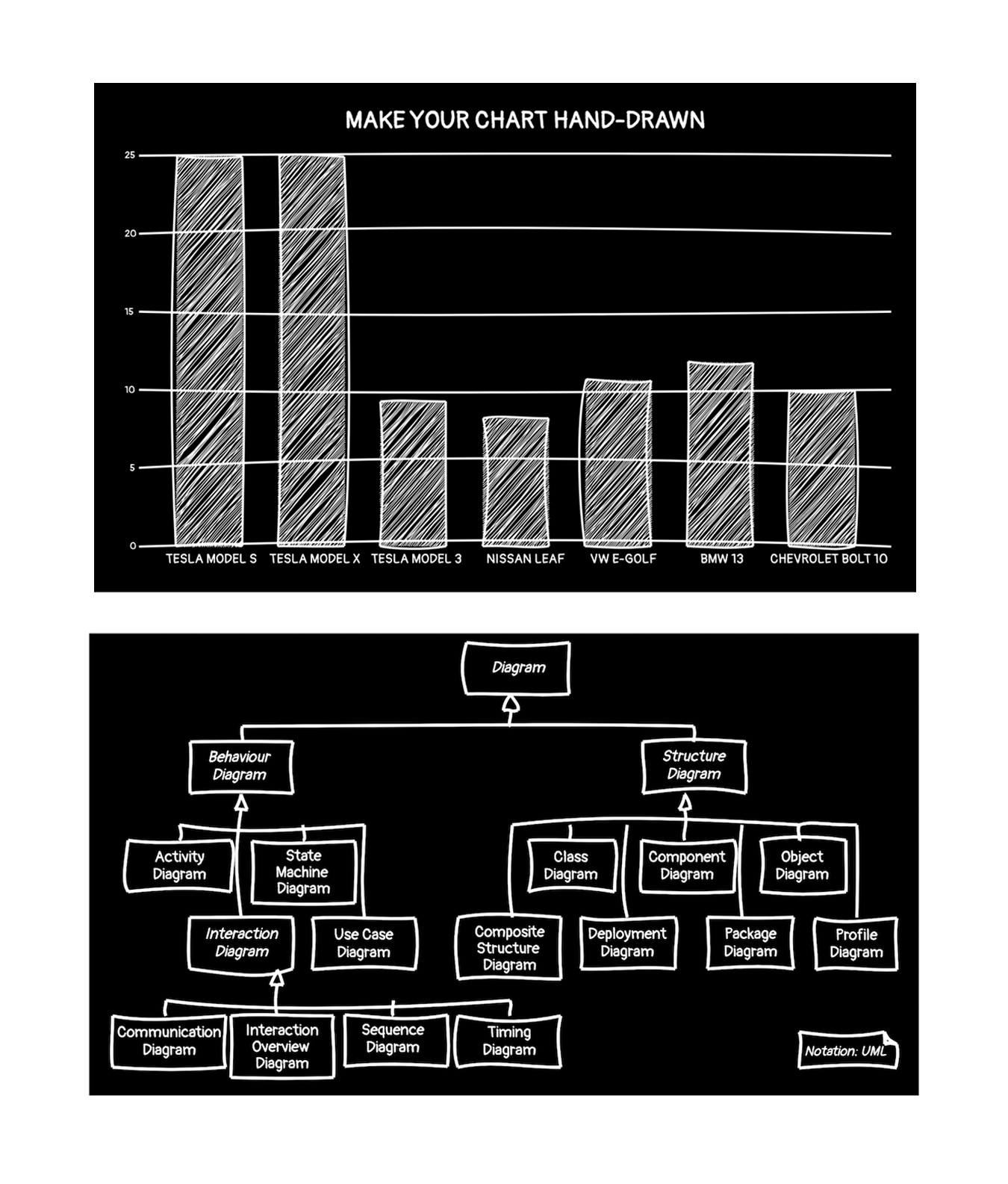
The workflow of instad.io is straight-forward: start from a given root DOM element, find all SVG objects, then recursively find all child elements, read out the basic attributes of the child elements, and use roughjs to create a hand-drawn style copy of the element, hiding the original element. This way the hand-drawn style SVG element replaces the original graphic.